C # (Sharp)
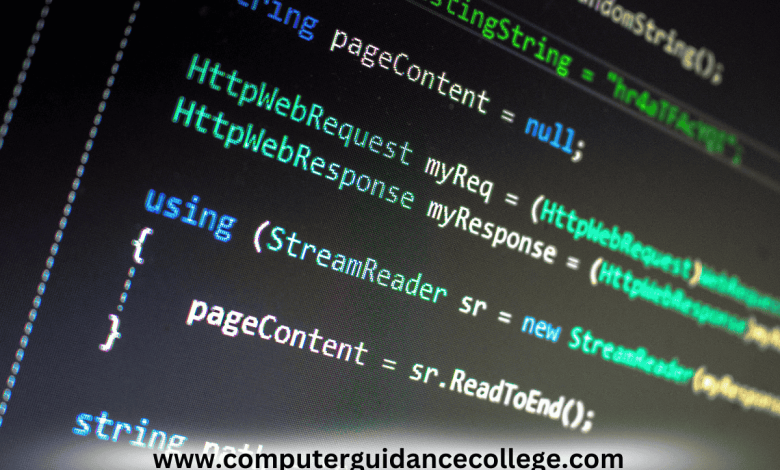
Introduction to C# Programming
Course Description:
This course is designed to provide a comprehensive introduction to the C# programming language, one of the most widely used languages in software development, particularly for building Windows applications, games (using Unity), web services, and mobile apps. Students will learn how to write efficient, scalable, and maintainable code, understand object-oriented programming (OOP) principles, and gain hands-on experience with key libraries and frameworks in the .NET ecosystem.
Course Objectives:
By the end of this course, students will be able to:
- Write basic and advanced C# programs.
- Understand and implement object-oriented programming (OOP) concepts like classes, inheritance, and polymorphism.
- Work with data types, collections, and LINQ for data manipulation.
- Build simple applications such as calculators, file I/O programs, and basic web APIs.
- Understand exception handling and debugging techniques.
- Use Visual Studio or Visual Studio Code for writing and testing C# code.
- Develop simple graphical user interface (GUI) applications using Windows Forms or WPF.
Course Modules:
Module 1: Introduction to C# Programming
- Overview of the C# language and .NET framework
- Setting up the development environment (Visual Studio or Visual Studio Code)
- Writing and running the first C# program
- Understanding the structure of a C# application
Module 2: Basic Programming Concepts
- Data types, variables, and constants
- Operators (arithmetic, relational, logical, etc.)
- Control structures (if-else, switch, loops)
- Arrays and basic collections (Lists, Arrays)
Module 3: Object-Oriented Programming (OOP) in C#
- Understanding Classes and Objects
- Constructors and Destructors
- Encapsulation and Access Modifiers
- Inheritance, Polymorphism, and Abstraction
- Interfaces and Abstract Classes
Module 4: Advanced Features and Techniques
- Delegates, Events, and Lambda Expressions
- Error handling and Exception management
- File I/O and working with streams
- Working with Collections (List, Dictionary, Stack, Queue)
- LINQ (Language Integrated Query)
Module 5: Creating Applications with C#
- Building a basic console application (e.g., a calculator)
- Developing a simple GUI application using Windows Forms
- Basics of building a web API with ASP.NET
- Using databases with C# (CRUD operations)
Module 6: Debugging, Testing, and Best Practices
- Debugging techniques in Visual Studio
- Unit testing and Test-Driven Development (TDD) basics
- Code refactoring and best practices
- Introduction to version control with Git
Assessment & Evaluation:
- Assignments: Weekly programming exercises (30%)
- Project: Develop a simple C# application (e.g., a calculator, to-do list app, or a simple game) (40%)
- Quizzes/Tests: Short tests to evaluate understanding of core concepts (20%)
- Final Exam: A comprehensive exam that covers all course material (10%)