Java
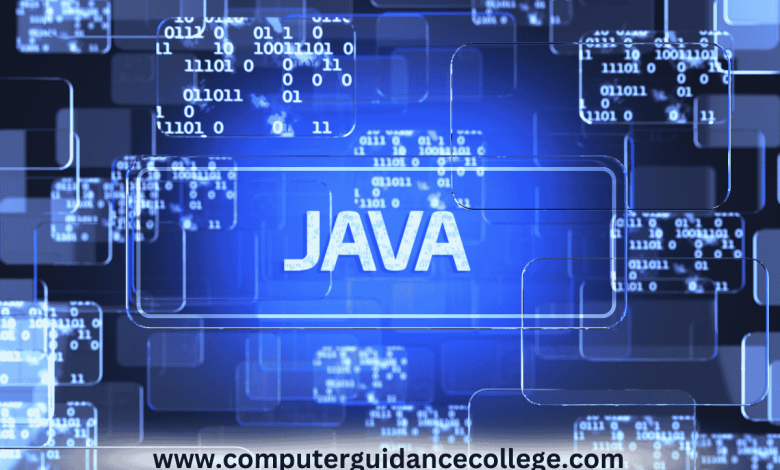
Introduction to Java Programming
Course Description:
This course introduces the core concepts and practices of programming in Java, a widely-used, high-level programming language. Students will develop a strong foundation in object-oriented programming (OOP), syntax, data structures, algorithms, and basic software development principles. By the end of this course, students will be capable of writing, debugging, and optimizing Java applications.
Course Objectives:
By the end of the course, students will be able to:
- Understand the core syntax and structure of Java programming.
- Write Java applications using object-oriented principles (encapsulation, inheritance, polymorphism).
- Implement common data structures such as arrays, lists, and maps.
- Work with fundamental algorithms and problem-solving techniques.
- Utilize Java’s libraries for input/output operations, error handling, and file management.
- Develop and debug Java programs with the help of integrated development environments (IDEs).
Course Modules:
Module 1: Introduction to Java
- History and features of Java
- Setting up the Java Development Kit (JDK)
- Introduction to Integrated Development Environments (IDEs)
- Writing the first Java program: “Hello, World!”
- Java syntax: variables, data types, operators, and control structures
Module 2: Object-Oriented Programming (OOP) Fundamentals
- Classes and objects in Java
- Methods and constructors
- Access modifiers (public, private, protected)
- Encapsulation and data hiding
- Introduction to inheritance and polymorphism
Module 3: Control Flow and Loops
- Conditional statements (if-else, switch)
- Looping structures (for, while, do-while)
- Break and continue statements
- Handling multiple conditions and exceptions
Module 4: Data Structures and Collections
- Arrays and multi-dimensional arrays
- Lists, sets, and maps from Java’s Collections Framework
- Iterating through collections
- Sorting and searching algorithms
Module 5: Exception Handling and File I/O
- Understanding exceptions and errors
- Try-catch blocks and custom exceptions
- File handling in Java (reading from and writing to files)
- Serialization and deserialization
Module 6: Java Development Tools and Best Practices
- Using debugging tools and breakpoints in IDEs
- Writing clean, readable code (comments, indentation, etc.)
- Unit testing with JUnit
- Refactoring and performance optimization
Module 7: Advanced Topics (Optional for Advanced Courses)
- Multithreading and concurrency in Java
- Working with databases (JDBC)
- Networking and Java’s socket programming
- Introduction to JavaFX for GUI development
Assessment & Evaluation:
- Assignments: Weekly programming exercises (40%)
- Midterm Exam: A practical coding exam (20%)
- Final Project: Building a simple Java application, demonstrating all learned concepts (30%)
- Participation and Quizzes: Ongoing quizzes and participation in discussions (10%)
Target Audience:
- Beginners who are new to programming
- Developers looking to switch to Java
- Students in computer science or software engineering courses
- Professionals interested in learning Java for backend or mobile development